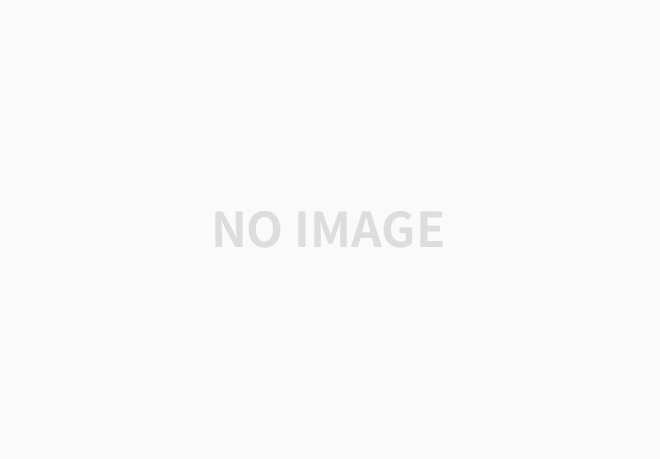
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
bool Sort(int a, int b)
{
return a > b; //내림차순 정렬
//return a < b; //오름차순 정렬
}
string solution(string s)
{
sort(s.begin(), s.end(), Sort);
return s;
}
|
문자열을 아스키 코드로 변환하면 대문자 A는 '65' 소문자 a는 '97'이기 때문에 내림차순 정렬만해주면 된다.
'코딩테스트 연습' 카테고리의 다른 글
코딩 테스트 - 문자열을 정수로 바꾸기 (0) | 2020.01.13 |
---|---|
코딩테스트 - 소수 찾기 (0) | 2020.01.13 |
코딩테스트 - 문자열 다루기 기본 (0) | 2020.01.13 |
코딩테스트 - 문자열 내 p와 y의 개수 (0) | 2020.01.10 |
코딩테스트 - 나누어 떨어지는 숫자 배열 (0) | 2020.01.10 |